HTML5テクニカルノート
CreateJS: ECMAScript 2015(ECMAScript 6)を用いて継承する
- ID: FN1710002
- Technique: HTML5 / CSS
- Library: EaselJS 1.0.0
CreateJSでクラスの継承をECMAScript 5で定めるときは、そのためにextend()
とpromote()
メソッドが用意されています。けれど、ECMAScript 2015(ECMAScript 6)では、標準の書き方でよいようです。
01 extend()とpromote()メソッドを使って継承する
メソッドextend()
はクラスを継承し、promote()
で親クラスのコンストラクタやオーバーライドされたメソッドが呼び出せるようになります。「CreateJS 14/12/12: 新たな継承の仕組みを定めるextend()とpromote()メソッド」には「例」として、つぎのコード001をご紹介しました。promote()
メソッドにより、親クラスのコンストラクタやオーバーライドされたメソッドは、「親クラス名_constructor」と「親クラス名_メソッド名」でそれぞれ呼び出せるようになります。
コード001■3次元座標のクラスから2次元座標のオーバーライドした距離のメソッドを呼出す
function Vector2D(x, y) {
this.Point_constructor(x, y);
}
createjs.extend(Vector2D, createjs.Point);
Vector2D.prototype.getLength = function() {
var square = this.x * this.x + this.y * this.y;
return Math.sqrt(square);
};
createjs.promote(Vector2D, "Point");
function Vector3D(x, y, z) {
this.Vector2D_constructor(x, y);
this.z = z;
}
createjs.extend(Vector3D, Vector2D);
Vector3D.prototype.getLength = function() {
var length2D = this.Vector2D_getLength();
square = length2D * length2D + this.z * this.z;
return Math.sqrt(square);
};
createjs.promote(Vector3D, "Vector2D");
var vector = new Vector3D(1, 1, 1);
console.log(vector.getLength()); // 1.7320508075688774
02 ECMAScript 6の構文でクラスを継承する
ECMAScript 6の構文でクラスを定めると、extend()
とpromote()
メソッドは使わずに済みます。クラスはclass
宣言し、extends
キーワードで継承します。コンストラクタをconstructor
メソッドとして定めたら、親コンストラクタの呼び出しに用いるのはsuper
キーワードです。クラスのメソッドにfunction
キーワードは添えません。変数のvar
宣言は、ECMAScript 6でも使えます。けれどできるだけ、ブロックスコープをもつ定数のconst
かlet
宣言に替えた方がよいでしょう。なお、クラスは関数(function
)と異なり、class
宣言する前には参照できないことにご注意ください(「ホイスティング(巻き上げ)」)。
// function Vector2D(x, y) { class Vector2D extends createjs.Point { constructor(x, y) { // this.Point_constructor(x, y); super(x, y); } // Vector2D.prototype.getLength = function() { getLength() { // var const square = this.x * this.x + this.y * this.y; return Math.sqrt(square); } // ; } // createjs.extend(Vector2D, createjs.Point); // createjs.promote(Vector2D, "Point");
サブクラス(Vector3D)から、オーバーライドしたスーパークラス(Vector2D)のメソッドを呼び出すときにもsuper
キーワードが使えます。このようにして、ECMAScript 6の構文では、extend()
とpromote()
メソッドを用いることなくクラスと継承が定められるのです。書き改めたスクリプトは、以下のコード002にまとめました。
class Vector2D extends createjs.Point { getLength() { const square = this.x * this.x + this.y * this.y; return Math.sqrt(square); } } class Vector3D extends Vector2D { getLength() { const length2D = super.getLength(); const square = length2D * length2D + this.z * this.z; return Math.sqrt(square); } }
コード002■ECMAScritp 6の構文で定めたクラスから継承したコンストラクタとメソッドを呼び出す
class Vector2D extends createjs.Point {
constructor(x, y) {
super(x, y);
}
getLength() {
const square = this.x * this.x + this.y * this.y;
return Math.sqrt(square);
}
}
class Vector3D extends Vector2D {
constructor(x, y, z) {
super(x, y);
this.z = z;
}
getLength() {
const length2D = super.getLength();
const square = length2D * length2D + this.z * this.z;
return Math.sqrt(square);
}
}
const vector = new Vector3D(1, 1, 1);
console.log(vector.getLength()); // 1.7320508075688774
03 ECMAScript 6のクラスと継承を使った作例
ご参考までに、ECMAScript 6のクラスと継承を使ったjsdo.itの作例も掲げましょう。Bitmap
クラスを継承したたくさんの粒子のオブジェクト(Particle)がマウスポインタの位置に合わせてアニメーションします。CreateJS 1.0.0に備わったStageGL
クラスを用いて、描画を滑らかにしました(「CreateJS 1.0.0のStageGLでStageを置き替える」の「処理を速める」参照)。
サンプル001■EaselJS 1.0.0 + ES6: Bursting particles animation with StageGL
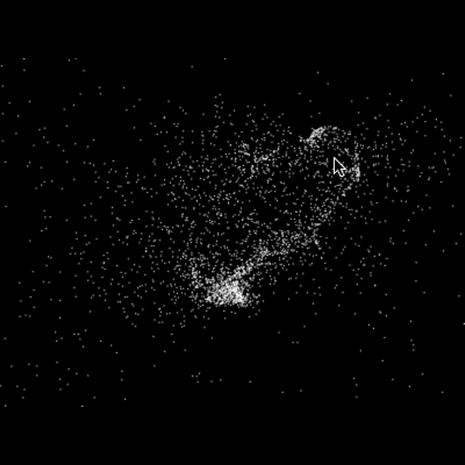
>> jsdo.itへ
04 ECMAScript 6の新しい数学的構文
本稿では、クラスと継承の定めがおもなお題でした。おまけとして、今回のコードで使えそうなECMAScript 6の新しい数学的構文をふたつご紹介します。ひとつは、数値の累乗です。Math.pow()
メソッドのほかに、べき乗演算子**
が加わりました。
// const square = this.x * this.x + this.y * this.y; const square = this.x ** 2 + this.y ** 2;
もうひとつ、2乗和の平方根を求めるメソッドがMath.hypot()
として備わっています(「hypotenuse」の意)。
getLength() { // const square = this.x ** 2 + this.y ** 2; return Math.hypot(this.x, this.y); // Math.sqrt(square); }
しかも、Math.hypot()
メソッドの引数は、3つ以上でも構いません。
class Vector3D extends Vector2D { getLength() { // const length2D = super.getLength(); // const square = length2D * length2D + this.z * this.z; return Math.hypot(this.x, this.y, this.z); // Math.sqrt(square); } }
作成者: 野中文雄
作成日: 2017年10月12日
Copyright © 2001-2017 Fumio Nonaka. All rights reserved.